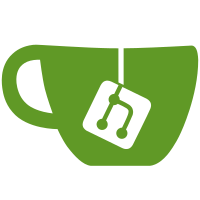
2 changed files with 100 additions and 20 deletions
@ -1,24 +1,96 @@ |
|||||
|
# -*- coding: utf-8 -*- |
||||
|
|
||||
import autocomplete_light |
import autocomplete_light |
||||
from models import Skola |
|
||||
|
|
||||
# This will generate a PersonAutocomplete class |
from models import Skola, Resitel, Problem |
||||
autocomplete_light.register(Skola, |
|
||||
# Just like in ModelAdmin.search_fields |
|
||||
search_fields=['nazev', 'mesto', 'ulice'], |
class SkolaAutocomplete(autocomplete_light.AutocompleteModelBase): |
||||
|
|
||||
|
model = Skola |
||||
|
|
||||
|
search_fields=['nazev', 'mesto', 'ulice'] |
||||
|
|
||||
|
split_words = True |
||||
|
|
||||
|
limit_choices = 15 |
||||
|
|
||||
|
attrs={ |
||||
|
# This will set the input placeholder attribute: |
||||
|
'placeholder': u'Škola', |
||||
|
# This will set the yourlabs.Autocomplete.minimumCharacters |
||||
|
# options, the naming conversion is handled by jQuery |
||||
|
'data-autocomplete-minimum-characters': 1, |
||||
|
} |
||||
|
|
||||
|
widget_attrs={ |
||||
|
'data-widget-maximum-values': 15, |
||||
|
'class': 'modern-style', |
||||
|
} |
||||
|
|
||||
|
autocomplete_light.register(SkolaAutocomplete) |
||||
|
|
||||
|
|
||||
|
class ResitelAutocomplete(autocomplete_light.AutocompleteModelBase): |
||||
|
|
||||
|
model = Resitel |
||||
|
|
||||
|
search_fields=['jmeno', 'prijmeni'] |
||||
|
|
||||
|
split_words = False |
||||
|
|
||||
|
limit_choices = 15 |
||||
|
|
||||
|
def choice_label(self, resitel): |
||||
|
return u"%s, %s (%s)" % (resitel.plne_jmeno(), resitel.mesto, resitel.rok_maturity) |
||||
|
|
||||
attrs={ |
attrs={ |
||||
# This will set the input placeholder attribute: |
# This will set the input placeholder attribute: |
||||
'placeholder': 'Skola', |
'placeholder': u'Řešitel', |
||||
# This will set the yourlabs.Autocomplete.minimumCharacters |
# This will set the yourlabs.Autocomplete.minimumCharacters |
||||
# options, the naming conversion is handled by jQuery |
# options, the naming conversion is handled by jQuery |
||||
'data-autocomplete-minimum-characters': 1, |
'data-autocomplete-minimum-characters': 1, |
||||
}, |
} |
||||
# This will set the data-widget-maximum-values attribute on the |
|
||||
# widget container element, and will be set to |
|
||||
# yourlabs.Widget.maximumValues (jQuery handles the naming |
|
||||
# conversion). |
|
||||
widget_attrs={ |
widget_attrs={ |
||||
'data-widget-maximum-values': 15, |
'data-widget-maximum-values': 15, |
||||
# Enable modern-style widget ! |
# Enable modern-style widget ! |
||||
'class': 'modern-style', |
'class': 'modern-style', |
||||
}, |
} |
||||
) |
|
||||
|
autocomplete_light.register(ResitelAutocomplete) |
||||
|
|
||||
|
|
||||
|
class ProblemAutocomplete(autocomplete_light.AutocompleteModelBase): |
||||
|
|
||||
|
model = Problem |
||||
|
|
||||
|
search_fields=['nazev'] |
||||
|
|
||||
|
split_words = False |
||||
|
|
||||
|
limit_choices = 10 |
||||
|
|
||||
|
def choice_label(self, p): |
||||
|
if p.stav == Problem.STAV_ZADANY: |
||||
|
return u"%s (%s, %s.%s)" % (p.nazev, p.typ, p.cislo_zadani.rocnik.rocnik, p.kod_v_rocniku()) |
||||
|
else: |
||||
|
return u"%s (%s, %s)" % (p.nazev, p.typ, p.stav) |
||||
|
|
||||
|
attrs={ |
||||
|
# This will set the input placeholder attribute: |
||||
|
'placeholder': u'Řešitel', |
||||
|
# This will set the yourlabs.Autocomplete.minimumCharacters |
||||
|
# options, the naming conversion is handled by jQuery |
||||
|
'data-autocomplete-minimum-characters': 1, |
||||
|
} |
||||
|
|
||||
|
widget_attrs={ |
||||
|
'data-widget-maximum-values': 10, |
||||
|
# Enable modern-style widget ! |
||||
|
'class': 'modern-style', |
||||
|
} |
||||
|
|
||||
|
autocomplete_light.register(ProblemAutocomplete) |
||||
|
|
||||
|
|
||||
|
Loading…
Reference in new issue